Dimensions
You can add Dimensions to your data items. Any property string that does not start with ‘$’ (reserved for metric key identifiers) and is not equal to ‘date’ or ‘unit’ will be recognized as a dimension.
Pro Tip: If you send one Dimension with multiple metrics, the dimension will be added to each metric.
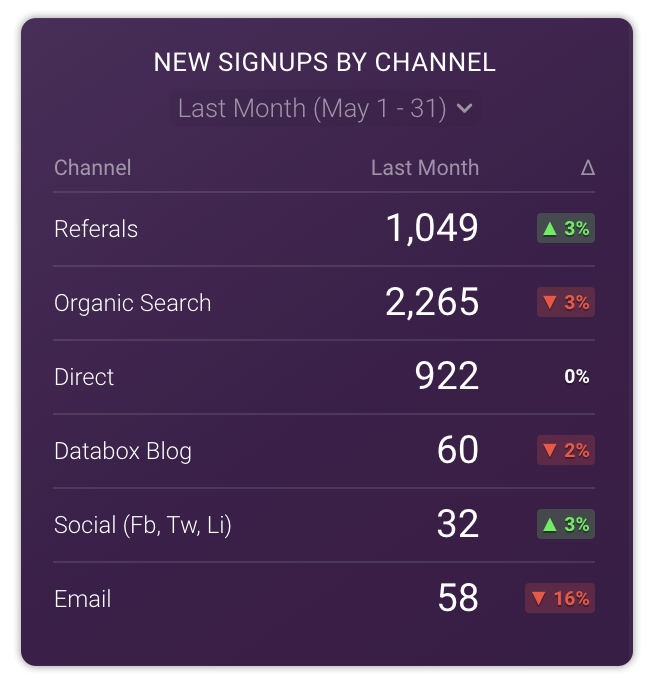
In Databox, you can filter and visualize your data for specific dimensions. That means you could view only retail sales, or compare sales across different channels. You can add as many dimensions as you’d like to each metric.